
#import "UIKit/UIKit.h"
@interface FirstViewController : UIViewController {
IBOutlet UILabel *opened;
IBOutlet UILabel *removed;
IBOutlet UILabel *reinstalled;
}
@property (nonatomic,retain) IBOutlet UILabel *opened;
@property (nonatomic,retain) IBOutlet UILabel *removed;
@property (nonatomic,retain) IBOutlet UILabel *reinstalled;
@end
FirstViewController.m make sure to synthesize and dealloc
#import "FirstViewController.h"
@implementation FirstViewController
@synthesize opened;
@synthesize removed;
@synthesize reinstalled;
- (void)dealloc {
[opened dealloc];
[removed dealloc];
[reinstalled dealloc];
[super dealloc];
}
@end
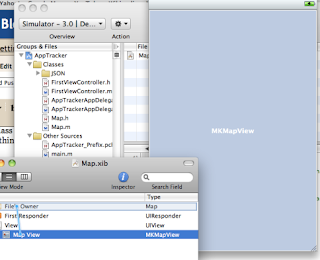
#import "UIKit/UIKit.h"
#import "MapKit/MapKit.h"
@interface Map : UIViewController {
IBOutlet MKMapView *map;
}
@property (nonatomic,retain) IBOutlet MKMapView *map;
@end
#import "Map.h"
@implementation Map
@synthesize map;
- (void)viewDidLoad{
//enable location on the map
[mapview setShowsUserLocation:YES];
[super viewDidLoad];
}
- (void)dealloc {
[map dealloc];
[super dealloc];
}
@end
[[UIApplicatio sharedApplication] registerForRemoteNotificationTypes:UIRemoteNotificationTypeBadge];
- (void)application:(UIApplication *)app didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)devToken;
- (void)application:(UIApplication *)app didFailToRegisterForRemoteNotificationsWithError:(NSError *)err;
#import "AppTrackerAppDelegate.h"
@implementation AppTrackerAppDelegate
@synthesize window;
@synthesize tabBarController;
- (void)applicationDidFinishLaunching:(UIApplication *)application {
[[UIApplication sharedApplication] registerForRemoteNotificationTypes:UIRemoteNotificationTypeBadge];
[window addSubview:tabBarController.view];
}
- (void)sendProviderDeviceToken:(const void*)devToken{
}
//Delegate methods that tell you if you succeeded.
- (void)application:(UIApplication *)app didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)devToken {
const void *devTokenBytes = [devToken bytes];
self.registered = YES;
[self sendProviderDeviceToken:devTokenBytes]; // custom method
}
- (void)application:(UIApplication *)app didFailToRegisterForRemoteNotificationsWithError:(NSError *)err {
NSLog(@"Error in registration. Error: %@", err);
}
@end
#include "CFNetwork/CFNetwork.h"
#include "sys/socket.h"
#include "netinet/in.h"
#include "arpa/inet.h"
#define PORT 2048
#define IPADDR "127.0.0.1"
//this is the callback method that gets called when
//client opens up the connection to the server
//if you were using more than one callback type you would need
//to check before continuing but since we only
//used the connect callback there was no need.
void ConnectCallBack(CFSocketRef socket, CFSocketCallBackType type,
CFDataRef address, const void *data, void *info){
//gets the native socket its a BSD socket so
//all normal BSD socket methods work
//like send you need to close the socket
CFSocketNativeHandle sock = CFSocketGetNative(socket);
send(sock, info, sizeof(info)+1, 0);
close(sock);
}
//this will open up a socket to our server
//we use the connectcallback to know when the socket actually connects
//once connected you need to have ConnectCallBack write the
//devToken to the server.
- (void)sendProviderDeviceToken:(const void*)devToken{
//socket reference and context, which get past around in the callback method
CFSocketRef sock;
CFSocketContext context = { 0, &devToken, NULL, NULL, NULL };
//open socket with default allocator its a TCP socket in ipv_4
sock = CFSocketCreate(NULL, PF_INET, SOCK_STREAM, IPPROTO_TCP, kCFSocketConnectCallBack, (CFSocketCallBack)ConnectCallBack, &context);
//if can't create socket fail to send devtoken
if(sock == NULL){
NSLog(@"error");
}
else {
//put the address of your server and port
//port define above as well as ip address
struct sockaddr_in addr;
memset(&addr, 0, sizeof(addr));
addr.sin_len = sizeof(addr);
addr.sin_family = AF_INET;
addr.sin_port = htons(PORT);
addr.sin_addr.s_addr = inet_addr(IPADDR);
//create reference and connect to server
CFDataRef connectAddr = CFDataCreate(NULL, (unsigned char *)&addr, sizeof(addr));
CFSocketConnectToAddress(sock, connectAddr, -1);
//run the socket through run loop,
//if connects callback method is called
CFRunLoopSourceRef sourceRef = CFSocketCreateRunLoopSource(kCFAllocatorDefault, sock, 0);
CFRunLoopAddSource(CFRunLoopGetCurrent(), sourceRef, kCFRunLoopCommonModes);
CFRelease(sourceRef);
CFRunLoopRun();
}
}
Wouldn't you need a way to stop your run loop or does that happen on it's own when you close the sock?
ReplyDelete